~ 4 min
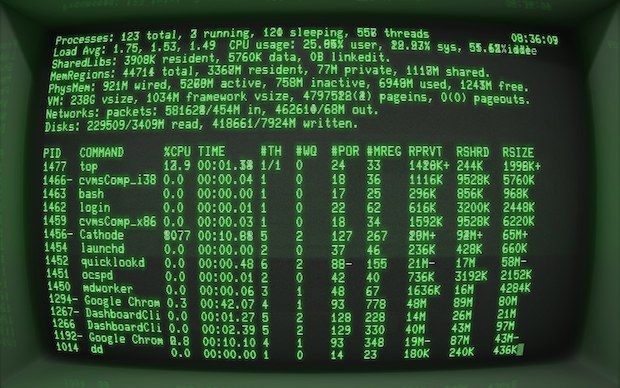
CMD Magic
Command Line Magic
Last year I published Vim Magic, where I gathered all the commands that I found particularly useful when using the Vim editor. I’ve kept updating it ever since whenever I’ve found new useful tricks. It’s been so far helpful to me as a reference and hopefully to somebody else out there too. This post is the same but for the command line in Linux/Unix.
This is not intended to be a tutorial with the basics for you to learn how to use the command line. Instead, it’s more like a reference for somebody who’s been using the terminal for some time now and wants to learn some new tricks or remember older ones.
Scripts
#!/bin/bash
use this as the first line of a bash script, for the interpreter to know how to handle it
Files
for i in *.png ; do convert "$i" "${i%.*}.jpg" ; done
convert all png files into jpgfind . -type f -exec grep -El "someWord|someOtherWord" {} \;
find all files in the current directory (and above) that containsomeWord
orsomeOtherWord
. An escaped semicolon is necessary to indicate the end ofexec
. The brackets are also necessary, since they contain the output of thefind
command, that will be fed into the grep commandfind . -type f | xargs grep -El "something|appstore"
exactly the same as the previous command, usingxargs
instead of-exec
find /path/to/files* -mtime +5 -exec rm {} \;
delete files older than 5 daysfind . -name '*.zip' -exec unzip {} \;
unzip all the zip files in the current directoryfind . -type d | egrep -o '.*src$'
findsrc
directories and none of their subdirectoriesfind . 2>/dev/null
find without showingpermission denied
messageswdiff one.txt two.txt | colordiff
color the differences between one.txt and two.txtdiff -rq(w) dir1 dir2
compare two directory structuresSOME_FILE=$(<some-file.txt)
load the contents of some-file.txt into the variable SOME_FILEcat oldfile.txt | tr -d '\n' > newfile.txt
create newfile.txt as a copy of oldfile where all newlines have been removedconvert file.{jpg,png}
equivalent toconvert file.jpg file.png
With xargs
you can avoid loops (it passes the stdout as input to the specified command):
find . -name *.png | xargs rm -rf
remove recursively all png filesgit branch --merged | grep -v \* | xargs git branch -D
remove all local git branches that have already been merged
Users and Groups
cat /etc/passwd | cut -d: -f1
list all userscat /etc/group | cut -d: -f1
list all groupschown user file
change the owner of a filechgrp group file
change the group of a filechown user:group file
change the owner and group of a file
Networking and SSH
lsof -nP | grep 9092
show which process is blocking the port 9092rsync -avz --remove-source-files -e ssh /local/dir remoteuser@remotehost:/remote/dir
SSH copy files and remove sourcessh user@your-server.com -L 2000:25
SSH session and port tunnelingssh -Y user@your-server.com
start an xtermsudo route delete default gw 10.0.2.2 eth0
remove the default gateway oneth0
sudo route add default gw 192.168.1.254 eth0
add the default gateway oneth0
wget -c -t 0 --timeout=60 --waitretry=60 --read-timeout=10 http://example.com/file.mkv
download the file using the partially downloaded file (resume-c
) retrying ad infinitum (-t 0
), with 60s timeout and 60s wait between attempts, resetting the connection if there’s no activity in 10 seconds (--read-timeout=10
)wget -r -np -nH -R index.html http://hostname/aaa/bbb/ccc/ddd/
download all files and subfolders of theddd
directory, recursively (-r
), without going to upper directories (-np
), not saving to the hostname folder (-nH
), excludeindex.html
files (-r index.html
)
Random Magic
<ctrl>+r
reverse search: in order to search for a command you previously usedcal
print the current monthsudo apt-get install build-essential checkinstall
in debian-based distros, install the essentials for building packages (make; make install)openssl base64 -in input.txt -out encoded.b64
encode input.txt in base64 and save it to the file encoded.b64sudo !!
run the last command with sudo- ` `+any command: it won’t go to the history
$( command )
it gives the output of commandservice --status-all
status of servicesecho "hello world" | sed -e "s/e/o/g" | sed -e "s/lo/a/g" | sed -e "s/world/mundo/g"
translate “hello world” to spanishdocker ps | awk '{print $8}'
print only the names of the current containers
Remote SSH session without a password
ssh-keygen -t rsa -b 2048
# Generate an rsa key pairssh-copy-id youruser@yourserver
# Authorize your key in the remote serverssh youruser@yourserver
# Login without entering a password